#301 BLE SiliconLabs Serial Brick
Overview
SiliconLabs BLE113を使用したBLE(Bluetooth Low Energy)のBrickです。
シリアルにて制御できるFirmwareが書き込まれているため、Arduino等からシリアル通信にてBLEを制御することができます。
BLEの転送レートは9600bpsに設定してあります。
接続
Serialコネクタへ接続します。
Serialコネクタは、Arduino用、RaspberryPI用、Ichigojam用のOUT/INシールドでは1箇所のみとなります。
写真はArduinoの接続例です。
写真はRaspberry Piの接続例です。
Support
Arduino |
RaspberryPI |
IchigoJam |
◯ |
◯ |
× |
BLE113 Datasheet
回路図
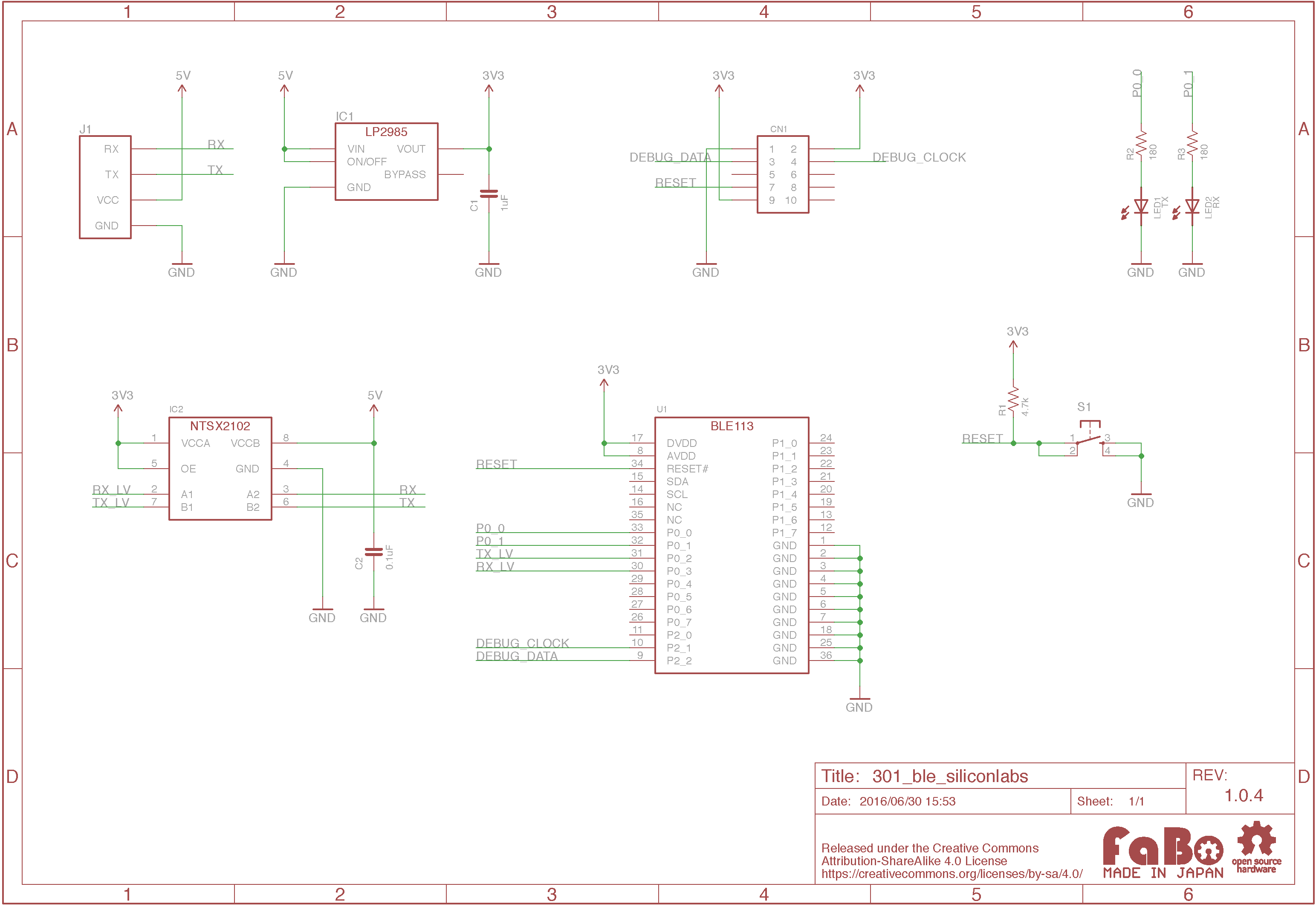
Library
for Arduino
ライブラリ名:「FaBoBLE-BLE113」
for RapberryPI
ライブラリ名:「FaBoBLE_BLE113」
Sample Code
for Arduino(Advertise)
SerialコネクタにBLE Brickを接続し、BLEを他の端末から接続できる状態(Advertise)にします。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46 | //
// FaBo Brick Sample
//
// brick_serial_ble
//
#include <SoftwareSerial.h>
SoftwareSerial bleShield(12, 13);
long previousMillis = 0;
long interval = 1000;
void setup()
{
// BLEとの通信用
bleShield.begin(9600);
// ログ出力用
Serial.begin(9600);
Serial.write("start!");
}
void loop()
{
// 一定時間ごとにコマンド実行
unsigned long currentMillis = millis();
if(currentMillis - previousMillis > interval) {
Serial.write("*\n");
previousMillis = currentMillis;
// アドバタイズ開始 (026100が返って来れば成功、BLEを検索すると見つかります)
// bleShield.write((byte)0x06); // パケットモードのみ
bleShield.write((byte)0x00);
bleShield.write((byte)0x02);
bleShield.write((byte)0x06);
bleShield.write((byte)0x01);
bleShield.write((byte)0x02);
bleShield.write((byte)0x02);
}
// 返答を出力
while (bleShield.available()) {
Serial.print(bleShield.read(), HEX);
}
}
|
for Arduino(Scan)
SerialコネクタにBLE Brickを接続し、他のBLE機器をスキャンしてシリアルモニタに出力します。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65 | //
// FaBo Brick Sample
//
// brick_serial_ble_scan
//
#include <SoftwareSerial.h>
SoftwareSerial bleShield(12, 13);
long previousMillis = 0;
long interval = 1000;
void setup()
{
// BLEとの通信用
bleShield.begin(9600);
// ログ出力用
Serial.begin(9600);
Serial.write("start!");
}
void loop()
{
// 一定時間ごとにコマンド実行
unsigned long currentMillis = millis();
if(currentMillis - previousMillis > interval) {
Serial.write("*\n");
previousMillis = currentMillis;
// 検索開始 (02620が返って来れば成功、BLEを検索します。)
// bleShield.write((byte)0x05); //パケットモードのみ
bleShield.write((byte)0x00);
bleShield.write((byte)0x01);
bleShield.write((byte)0x06);
bleShield.write((byte)0x02);
bleShield.write((byte)0x02);
delay(1000);
}
int count = 0;
// 返答を出力
while (bleShield.available()) {
if (count==5){
Serial.write('\n');
}
Serial.print(bleShield.read(), HEX);
count++;
}
delay(100);
// 検索終了 (02640が返って来れば成功、検索を終了します)
// bleShield.write((byte)0x04); // パケットモードのみ
bleShield.write((byte)0x00);
bleShield.write((byte)0x00);
bleShield.write((byte)0x06);
bleShield.write((byte)0x04);
delay(100);
// 返答を出力
Serial.write('\n');
while (bleShield.available()) {
Serial.print(bleShield.read(), HEX);
}
Serial.write('\n');
}
|
Parts
- SiliconLabs BLE113 BluetoothLE ModuleIC
GitHub
- https://github.com/FaBoPlatform/FaBo/tree/master/301_ble_siliconlabs